### The Eth1 part of the merge
---
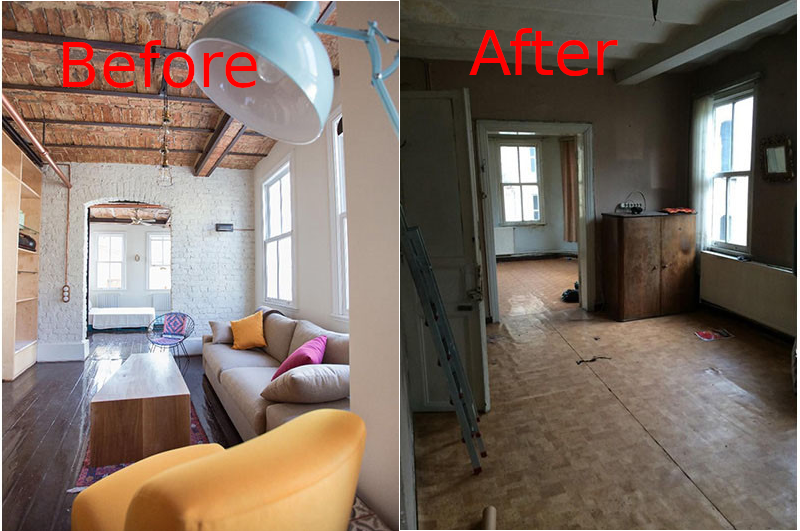
---
```go
type ExecutableData struct {
Coinbase common.Address
StateRoot common.Hash
GasLimit uint64
GasUsed uint64
Transactions []*types.Transaction
ReceiptRoot common.Hash
LogsBloom []byte
BlockHash common.Hash
ParentHash common.Hash
Difficulty *big.Int
}
```
---
### Changes so far
* Deactivate block reception in protocol
* Add RPCs calls (defined in `eth/eth2.go`)
* Add `BEACONBLOCKROOT` instruction
---
### Block production
```go
type ProduceBlockParams struct {
ParentHash common.Hash
RandaoMix common.Hash
Slot uint64
Timestamp uint64
RecentBeaconBlockRoots []common.Hash
}
```
---
### Block insertion
```go
type InsertBlockParams struct {
RandaoMix common.Hash
Slot uint64
Timestamp uint64
RecentBeaconBlockRoots []common.Hash
ExecutableData ExecutableData
}
```
---
#### `BEACONBLOCKROOT`
* Set at `0x48`
* Low gas price $G_{blockhash}$
* Copied from the EngineData
* Replace `BLOCKHASH` in the future?
---
#### Challenges & Gotchas
* block insertion can reorg based on difficulty
* might cause eth2 client and eth1 engine to differ on what the canonical chain is
* Gaps in the block number
* block number ≠ slot number + offset
* Follow the regular rules for difficulty
* (bomb, adaptative...)
---
### Upcoming changes
* Other things landing in eth1 (BLS, binary trees, stateless...)
* Better tests
* Merge
* Sync
---
#### Hive
* Multi-client test environment
* Docker-based
* Define a fork block and see how the transition works
* Step before testnet
---
#### Merge
* Like other forks: activate at block specified in config
* Deactivate mining but not the block production
* Disable block sealing in consensus engine :warning:
* Don't verify the block's difficulty
* Don't reorg for a higher difficulty :warning:
---
#### Block Sync (WIP)
* Inserted from the finalization point with `eth2_insertBlock`
* leave it to eth2 to make this work :shrug:
* problem: no verification from the start
* Download the header chain backwards until the genesis
* Require significant tweaks to the downloader
---
#### State Sync (WIP)
* Problem: state of a finalized block will could be garbage-collected
* Download the state at the last confirmed block
* Snap sync could be used for downloading the state
---
#### Thank you
 gballet
https://github.com/gballet/go-ethereum/tree/catalyst-for-executable-beacon-chain